Angular
Angular is a popular open-source web application framework maintained by Google and a community of developers. It is written in TypeScript and is widely used for building dynamic, single-page web applications (SPAs).
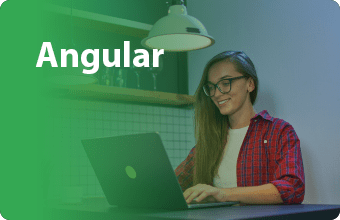
Angular is widely adopted in the development community for building modern, responsive, and scalable web applications. It’s important to note that there are different versions of Angular (e.g., AngularJS, Angular 2+, etc.), and each version has its features and conventions.
Here are some key points about Angular:
- TypeScript:
- Angular is built with TypeScript, which is a superset of JavaScript. TypeScript adds static typing to JavaScript, making it more scalable and maintainable for large projects.
- Modular Architecture:
- Angular applications are organized into modules, which are sets of cohesive components, services, and other code that work together. This modular architecture helps in organizing and scaling the application.
- Components:
- Components are the building blocks of Angular applications. They encapsulate the application’s functionality and UI, and they can be reused throughout the application.
- Directives:
- Angular provides directives to extend HTML with new attributes and behavior. Directives such as ngFor and ngIf are commonly used to manipulate the DOM based on data in the application.
- Services:
- Services encapsulate business logic, data manipulation, and communication with external services. They promote reusability and maintainability by providing a way to share functionality across components.
- Dependency Injection:
- Angular uses dependency injection to manage the components’ dependencies. This makes components more modular, testable, and easier to maintain.
- Two-Way Data Binding:
- Angular supports two-way data binding, meaning changes in the user interface automatically update the application state, and vice versa. This simplifies the synchronization of the model and the view.
- Routing:
- Angular includes a powerful routing system that enables the creation of single-page applications with multiple views. The Angular Router helps manage navigation within the application.
- Forms:
- Angular provides a robust set of tools for working with forms. Reactive Forms and Template-Driven Forms are two approaches for handling user input and form validation.
- Observables:
- Angular leverages Observables from the RxJS library to handle asynchronous operations, such as HTTP requests. Observables provide a convenient way to work with asynchronous data streams.
- Testing:
- Angular is designed with testability in mind. It comes with built-in support for testing using tools like Jasmine and Karma. This makes writing unit tests for components, services, and other parts of an Angular application easier.
- CLI (Command Line Interface):
- The Angular CLI provides a set of commands for creating, building, testing, and deploying Angular applications. It streamlines the development process and helps manage the project structure.
1. Introduction to Web Development and Angular (24 hours)
- Basics of HTML, CSS, and JavaScript
- Overview of Angular Framework
- Setting up the development environment
2. Angular Architecture and Components (32 hours)
- Understanding Angular modules and components
- Component lifecycle and hooks
- Data binding and interpolation
3. Directives and Pipes (24 hours)
- Using built-in directives
- Creating custom directives
- Understanding and creating custom pipes
4. Services and Dependency Injection (24 hours)
- Creating and using Angular services
- Understanding dependency injection
- Injectable services and providers
5. Forms in Angular (32 hours)
- Template-driven forms
- Reactive forms and form validation
- Handling form submissions
6. Routing in Angular (24 hours)
- Configuring Angular routing
- Route guards and navigation
- Lazy loading modules
7. HTTP Client and Observables (24 hours)
- Making HTTP requests
- Handling Responses with Observables
- Error handling and asynchronous programming
8. Testing in Angular (24 hours)
- Unit testing with Jasmine and Karma
- E2E testing with Protractor
- Writing testable Angular code
9. State Management with NgRx (32 hours)
- Introduction to NgRx and Redux pattern
- Actions, reducers, and selectors
- NgRx effects and asynchronous state management
10. Angular CLI and Build Optimization (16 hours)
- Using Angular CLI for project scaffolding
- Build optimization techniques
- Deployment strategies
11. Advanced Angular Features (40 hours)
- Animations in Angular
- Internationalization (i18n)
- Progressive Web App (PWA) features
- Server-Side Rendering (SSR)
12. Angular and Backend Integration (24 hours)
- Integrating Angular with backend services
- Authentication and authorization
- Handling security concerns
13. Angular Best Practices and Code Optimization (16 hours)
- Code structure and organization
- Performance optimization tips
- Accessibility considerations
14. Project Work and Real-world Applications (70 hours)
- Applying knowledge to real-world projects
- Building a complete Angular application
- Continuous integration and deployment practices
15. Exploring Angular Ecosystem (16 hours)
- Angular Material for UI components
- Third-party libraries and modules
- Staying updated with Angular releases
You May Like
Our thoughtfully designed internship programs provide a tailored and enriching experience for aspiring professionals.