Enhance PDF Generation Efficiency in Laravel
by Apsara Raj (Software Developer) | Updated on February 27, 2024
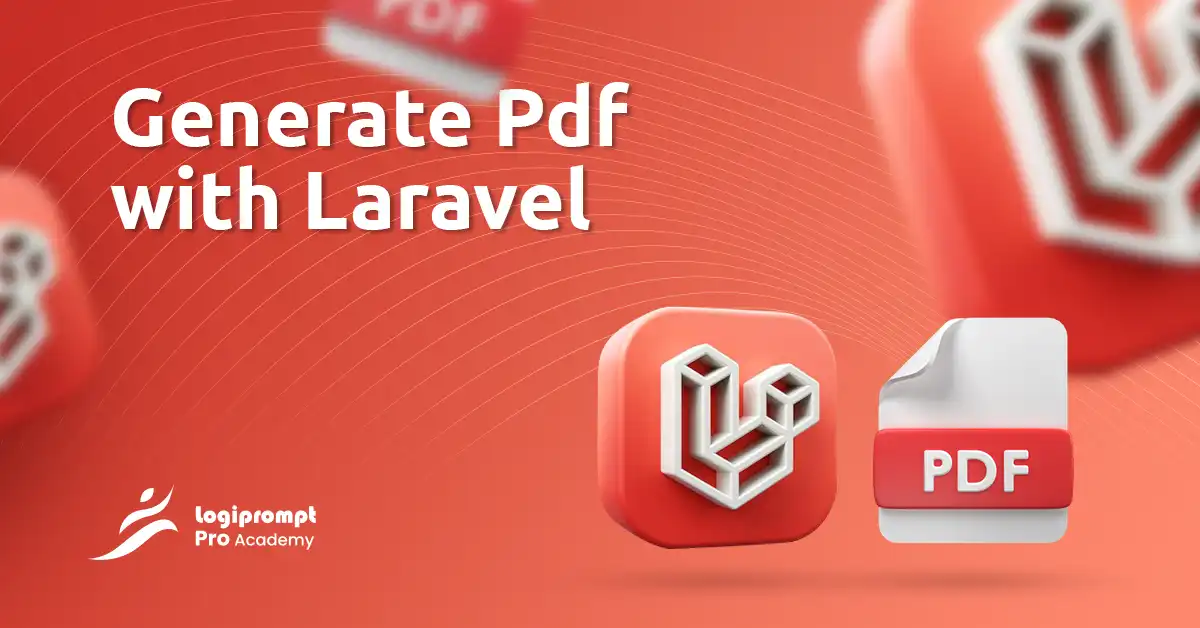
I’m generating pdf in Laravel but the issue is that sometimes data is saved in the database and pdf is not generated when I check the pdf folder there are no pdf files with that name, this does not happens every time I generate the PDF but there are some entries in my database for which the pdf is not generated. I referred to multiple sites to solve the problem, and finally, I found a website that provides step-by-step guidance for the entire process, which is
https://www.positronx.io. On this particular site, the instructions focus on downloading details for all users, whereas my requirement is to generate a PDF specifically for selected customers of my choice.
My objective is to generate PDFs selectively for chosen customers. To overcome this hurdle, I am exploring alternative strategies to tailor the PDF generation process to my specific requirements. This involves delving deeper into Laravel’s documentation and seeking insights from the Laravel community to pinpoint the root cause of the sporadic PDF generation issue and implement a targeted solution for the identified problem entries in the database.
In both pdf files I am facing major formatting issues. I have checked that the HTML of the bootstrap template is added correctly in the view file and when I echo it, it shows the correct HTML with proper formatting.Creating step-by-step procedures on paper can serve as a handy reference guide for you and others who may be working on the same task.
Below are the general steps for generating a PDF in Laravel
- First, create an interface with a structure similar to this. When clicking on “Invoice,” navigate to a page that displays the user details in a table format and Install DomPDF Library using the command composer requires barryvdh/laravel-dompdf
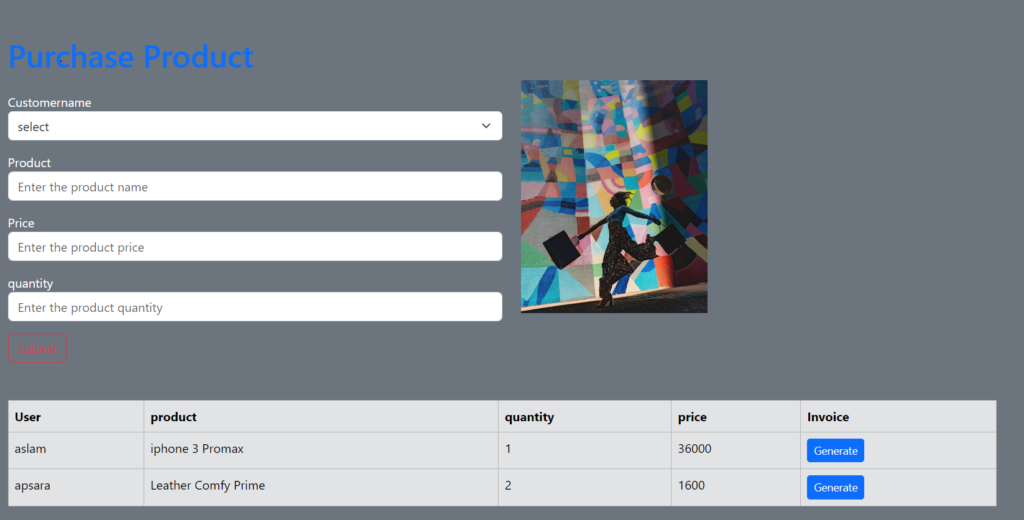
- Then Open the config/app.php file and incorporate the DomPDF service provider in the providers array along with the DomPDF facade to the aliases array.
'providers' => [
Barryvdh\DomPDF\ServiceProvider::class,
],
'aliases' => [
'PDF' => Barryvdh\DomPDF\Facade::class,
]
Ultimately, you can use the DomPDF to convert HTML to PDF File in Laravel using the following attribute.
Use PDF:
- Create a migration for store details of products,the code is given below
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*/
public function up(): void
{
Schema::create('purchasedetail', function (Blueprint $table) {
$table->increments('id');
$table->string('user');
$table->string('product');
$table->string('price');
$table->string('quantity');
$table->timestamps();
});
}
/**
* Reverse the migrations.
*/
public function down(): void
{
//
}
};
And for storing details I use this method in my controller
public function store(Request $req)
{
$details=new Purchasedetail();
$details->user=$req->user;
$details->product=$req->product;
$details->price=$req->price;
$details->quantity=$req->quantity;
$details->save();
return redirect()->back();
}
- Create corresponding routes.And my web.php is just look like this
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\PurchaseController;
/*
|------------------------------------------------------------------------
--
| Web Routes
|------------------------------------------------------------------------
--
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider and all of them will
| be assigned to the "web" middleware group. Make something great!
|
*/
Route::get('/',[PurchaseController::class,'index']);
Route::post('/store',[PurchaseController::class,'store'])->name('store');
Route::get('/downloadpdf/{id}',[PurchaseController::class,'downloadpdf'])
->name('downloadpdf');
Route::get('/loadpdf/pdf/{id}', [PurchaseController::class,
'createPDF'])->name('loadpdf');
- When clicking the invoice button, go to a page and display the details of that particular user. For i am using these methods
public function downloadpdf($id)
{
$allpdfdeatils=Purchasedetail::find($id);
return view('pdfdetails',compact('allpdfdeatils'));
}
public function createPDF(Request $request,$id) {
$data = Purchasedetail::find($id);
$user = $data['user'];
$product = $data['product'];
$price = $data['price'];
$quantity =$data['quantity'];
$alldata = [$user, $product, $price,$quantity];
$pdf = PDF::loadView('pdf_view', compact('alldata'));
return $pdf->download('invoice.pdf');
}
- I designed a page like this for viewing the user’s details.

- Designed a new page named pdf_view.blade.php.
You can design this page in your own way. Here I decided to display my pdf content as a list.
$pdf = PDF::loadView('pdf_view', compact('alldata'));
return $pdf->download('invoice.pdf');
These two lines have caused me numerous troubles and errors. I’m confused about the meaning of “pdf_view,” and I’m unsure what “invoice.pdf” represents. Finally, I’ve realized that “pdf_view” is likely a new view page designed to display all PDF contents, and “invoice.pdf” is likely the name given to the PDF file.
Conclusion
Whether you are a Laravel beginner seeking help in HTML-to-PDF conversion or an experienced PHP developer willing to convert simple HTML strings or data into a well-formatted PDF document, with this how-to guide, it becomes effortless to create dynamic and highly customizable PDF files in Laravel.
In this tutorial, you have learned how to generate portable document format (PDF) files in Laravel using the DomPDF library, with this package, you can easily convert hypertext markup language (HTML) content into downloadable PDF documents.
You can check the official documentation of DomPDF; plugin; It offers exclusive methods that can help you extend the functionalities PDF generation process in Laravel.
Explore & Learn
Embark on a journey of knowledge and discovery with our curated collection of articles, insights, and updates to foster continuous learning and exploration.